ISR pages are pre-rendered pages that become static once a user first visits them. The pages then stay static for a given period of time (as specified by the revalidate parameter, time-based revalidation). Recently it was introduced that a rebuild can also be triggered (on-demand revalidation). With ISR, you have a static website without having every page statically built during runtime.
Some of the disadvantages are that upon a new deployment, the very first request to an ISR page is going to be slow. Once the page is successfully generated though, the static file is saved in the cache. All later requests to this page will be as fast as a request to a static page.
New to Next.JS? Read our introductory guide to Next.JS and understand why Next.JS is suited for search engine optimisation. For more tips on how to improve your core web vitals read more here.
Table of Contents
- Why are people excited about ISR?
- When is Incremental static regeneration right for you?
- Large websites often require a hybrid solution of rendering methods
- How do I build an ISR page?
- What is ISR revalidation?
- How does time-based ISR work?
- How does on-demand ISR work?
- What do people mean by “warming up pages”?
Why are people excited about ISR?
With Next JS ISR, you have a static website without having every page statically built during runtime. For example, if you run a large e-commerce website with 10,000 product pages and you want to implement static site generation for your entire website, you will spend a lot of time and resources during the build process to do so. This can be bad for several reasons.
Why is it bad to statically build your entire pages during build time?
1.) It might not be feasible to statically build that many pages during build time. For example, at the time of writing, Vercel build time is limited to 45 minutes. You will not be able to statically generate that many pages (10,000) during build time.
2.) Data will not be fresh. If your website is data-heavy and you need to update some pages every 20 minutes, you would need to rebuild the whole website every 20 minutes. It is simply not feasible to statically build the entire site while ensuring the user has fresh data.
3.) Your cloud spending might go through the roof. Most likely, 99% of your traffic goes to 1% of your pages. Rebuilding that many pages for nothing on a regular basis will lead to unnecessary costs.
4.) Shipping a new version of your website will take forever. Since your build process is many hours, it will then take hours for users to see your new feature. Even worse, what if there’s a bug on your website? Waiting hours until your build is completed when users are experiencing bugs is not fun.
When is Incremental static regeneration right for you?
1.) If you run a large website and you want to have all pages statically generated. At the time of writing, Vercel build times out after 45 minutes of the building. You have to choose which pages you want to render statically and which pages are not. For example, you run a large e-commerce website.
2.) You would like the static page to be updated once in a while (e.g. every 10 minutes). This could be because new, fresh data is present. Specify the revalidate time and the site will be rebuilt
3.) You have a budget for it. If most of your pages are ISR and you revalidate them frequently, Vercel will charge you for this. For large websites, costs might go up to thousands of dollars per month.
Large websites often require a hybrid solution of rendering methods
ISR pages are great if you are building user-facing web pages which require no real-time data and you are striving to optimise your website for SEO. On a side note, large websites often require a hybrid solution of rendering methods. Let’s take the example of a large e-commerce website.
A combination of the following rendering methods can be used:
- Static: The About section is likely to never update and it is built statically.
- Server-side Rendering: The user logs in to his account and wants to see his basket. This page can be built using client-side rendering. The member area is not indexed with search engines and you want to ensure that the user sees the latest data.
- ISR: The individual product pages (e.g. ecommercewebsite.com/yellow-toothbrush-for-children) can be built using ISR. We want the page to rank for the term ‘Yellow toothbrush for children’ and to optimise our Core Web Vitals, we build it with ISR. Once a day, we also update the product description and title to see if more people click on the page. ISR with a revalidation time of 24 hours allows us to automatically rebuild the page every day in the background.
How do I build an ISR page?
There are a few components to building an ISR page. If you are new to Next.JS, read our in-depth guide on how to get started with Next.JS.
Fetching the data with getstaticprops function
Here, we assume that your ISR page data fetching. Using getStaticProps, you can fetch all your data and pass them on in the props.
Compared to server-side or client-side generation, it’s ok if getStaticProps is slow (for example, you might have an API call that takes 3 seconds). This would be a disaster for server-side or client-side rendering because the same 3-second API call will be performed every time someone requests this web page.
With ISR pages, the API endpoint will be called at the initial build of the ISR page. Should be page be revalidated, the page will be rebuilt in the background without the user being affected by it.
GetStaticProps also returns a revalidate parameter. The parameter specifies at which time interval the page is automatically rebuilt.
export const getStaticProps: GetStaticProps = async ctx => {
const coffeeDescription = await getCoffeeDescription();
const coffeeDescriptionText = JSON.stringify(coffeeDescription, null, 2)
await sleep(sleepingTime);
return {
props: {
coffee: coffeeDescriptionText
},
revalidate: 60 * 60 // Regenerate this page every hour.
}
}
Returning the components to be rendered with NextPage
The NextPage receives the props from getStaticProps and returns the element to be rendered.
const ISRpage: NextPage<IProps> = props => {
const { coffee } = props;
return (
<div>
<h1>ISR</h1>
<p>This page turns into a static page and is regenerated every N seconds.</p>
<pre>{coffee}</pre>
</div>
)
}
Putting it all together, an ISR page can look like this:
import React from 'react'
import { GetStaticProps, NextPage } from "next";
import { getCoffeeDescription } from '@/api/api';
import { sleep } from '@/utils/index'
import sleepingTime from '@/common/constants'
interface IProps {
coffee: string;
}
const ISRpage: NextPage<IProps> = props => {
const { coffee } = props;
return (
<div>
<h1>ISR</h1>
<p>This page turns into a static page and is regenerated every N seconds.</p>
<pre>{coffee}</pre>
</div>
)
}
export const getStaticProps: GetStaticProps = async ctx => {
const coffeeDescription = await getCoffeeDescription();
const coffeeDescriptionText = JSON.stringify(coffeeDescription, null, 2)
await sleep(sleepingTime);
return {
props: {
coffee: coffeeDescriptionText
},
revalidate: 60 * 60 // Regenerate this page every hour.
}
}
export default ISRpage
For a real-world example, please refer to https://playground.seomastered.co/rendering/singlePage/isr
What is ISR revalidation?
ISR pages can be revalidated (i.e. new data is fetched and the cached version of the page will be overwritten with a new page with fresh data). The deletion of the cache and rebuilding of the website is done in the background and do not affect the page speed of the user. There are two ways how to revalidate the ISR page (i.e. update static pages):
How does time-based ISR work?
With time-based revalidation, the ISR page will be revalidated (i.e. fresh data will be fetched) every N seconds. You can add the revalidate prop to getStaticProps to rebuild the web page every N seconds. Rebuilding and revalidating mean that fresh data is loaded (in the background), a new HTML page is built and the old page is deleted from the cache and replaced with the new HTML.
How does on-demand ISR work?
On-demand revalidation means that you can trigger revalidation of a specific page yourself. For example, if you run a job portal and an employer has just updated their job post, you can regenerate the web page for that particular web page. Basically, you send a request to this URL.
https://<your-site.com>/api/revalidate
The path that you specify in the request, will then be rebuilt.
// pages/api/revalidate.js
export default async function handler(req, res) {
await res.revalidate('/path-in-your-website-to-revalidate')
return res.json({ revalidated: true })
}
What do people mean by “warming up pages”?
The first request to a ‘cold’ ISR page will trigger the generation of the static HTML. This can take time. In order to reduce the initial load time of the ISR page, people will trigger a request to all ISR pages to ‘warm them up’. After the initial request (triggered by you), the HTML is built and the page is static.
This is a simple script to warm up your pages. For large pages, this might be too slow and you need to parallelise it. Be careful though to not flood your website with too many requests.
import time
list_of_your_isr_pages = []
for current_page in list_of_isr_pages:
response = requests.get(url, headers={
'User-Agent': 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_9_3) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/35.0.1916.47 Safari/537.36'
})
time.sleep(0.1)
One ‘hack’ to avoid warming up too many (statically generated pages, never-visited) pages is to find all your most visited pages in Google Search Console. You can export the most frequently visited pages from Google Search Console and download them and put them into a list (see list_of_your_isr_pages). All pages will then be warming up and the static files will be served to the user.
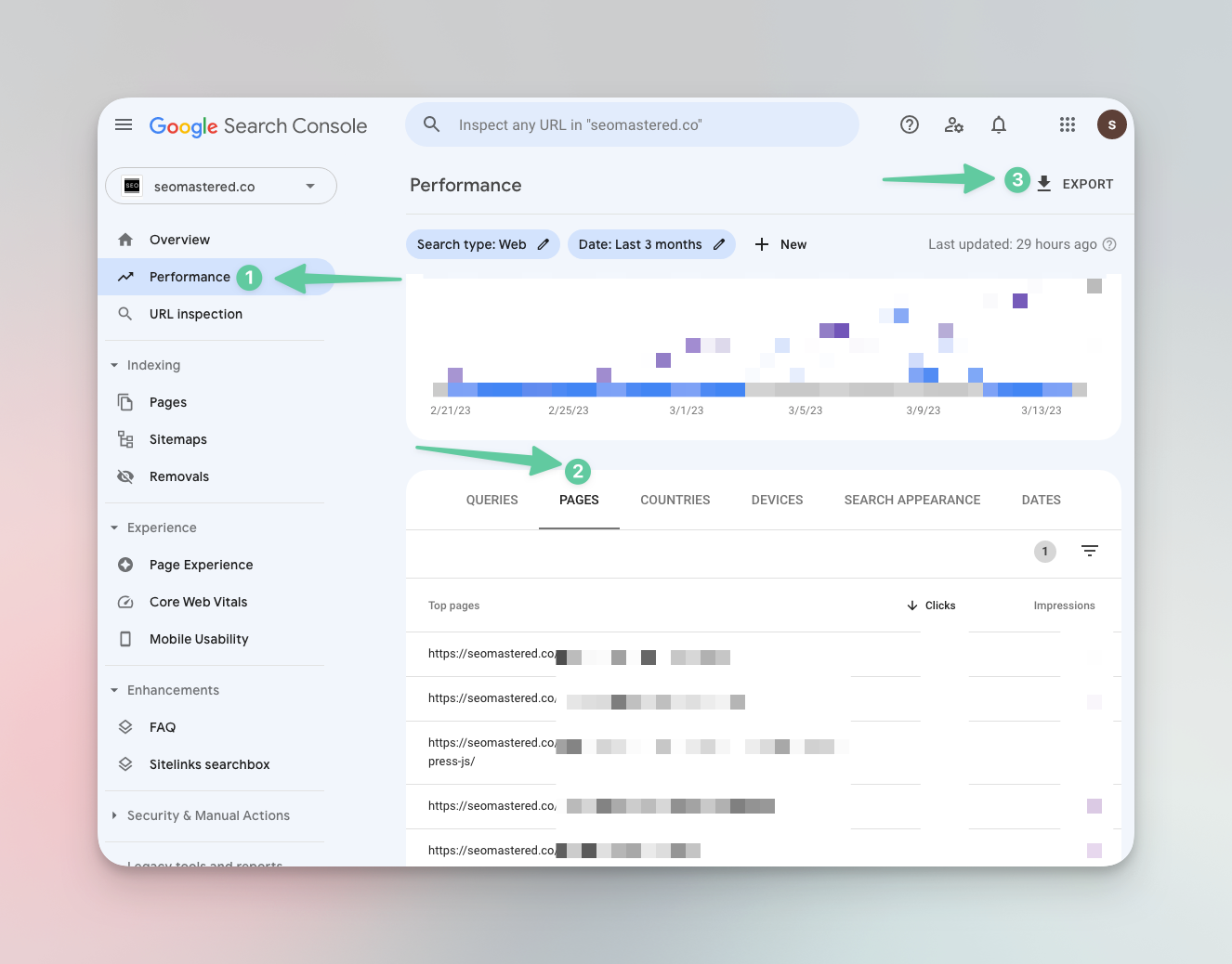